.NET Authentication/Authorization with PropelAuth
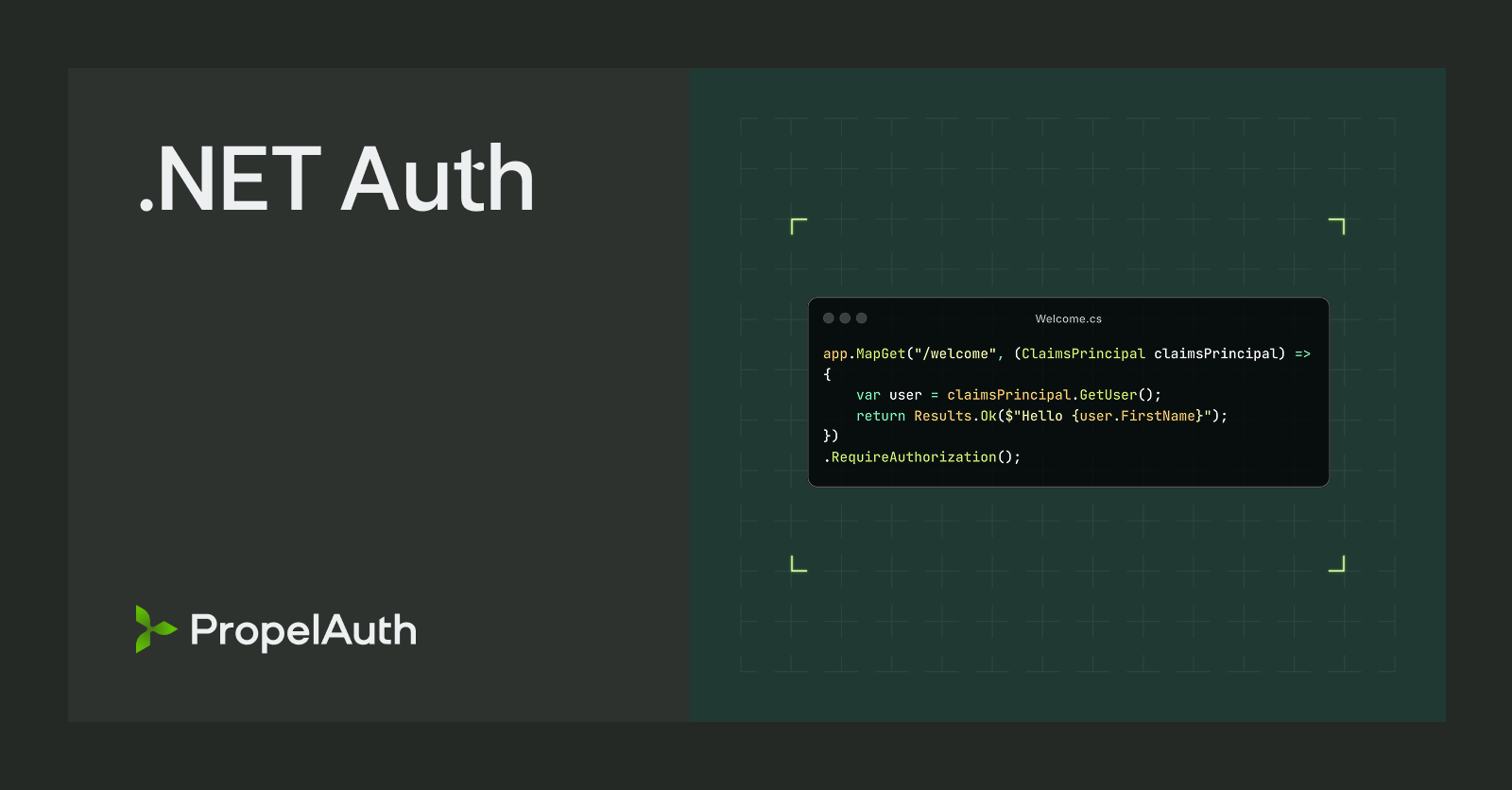
Want to skip straight to it? See our reference documentation, our full-stack documentation (for Blazor), or an example app build with Blazor server.
.NET is a powerful and versatile platform for building secure, high-performance applications. Thanks to its built-in claims-based identity system, it's a breeze to add authentication and authorization to your backend.
And while .NET’s built in authentication is powerful, it’s also relatively complex. At PropelAuth, we've always aimed to provide a straightforward way to integrate authentication into any tech stack. We’re thrilled to announce our first-class support for .NET.
Protecting Routes
Protecting a backend route is as simple as:
app.MapGet("/welcome", (ClaimsPrincipal claimsPrincipal) =>
{
var user = claimsPrincipal.GetUser();
return Results.Ok($"Hello user with ID {user.userId}");
})
.RequireAuthorization();
The User
class has first class concepts for organizations/tenants as well as roles and permissions:
app.MapGet("/org/{orgId}/adminonly", (ClaimsPrincipal claimsPrincipal, string orgId) =>
{
var user = claimsPrincipal.GetUser();
var userInOrg = user.GetOrg(orgId);
if (userInOrg.IsRole("Admin"))
{
return Results.Ok($"You are an Admin in {userInOrg.orgName}");
} else {
return Results.Forbid();
}
})
.RequireAuthorization();
Protecting pages that for server-rendered Blazor applications uses Blazor’s built in @attribute [Authorize]
.
Initializing the library will take care of setting up one or both of Bearer token authentication (for APIs) or OAuth authentication (for full stack applications).
Ready to get started?
With PropelAuth, you can configure login and signup pages, account management, organization/tenant features, MFA, user impersonation, and enterprise SSO —all in just a few minutes. We support a wide range of frontends, from React to Blazor, so you can mix and match .NET on the server with practically any UI.
And best of all, getting started is easy. Check out our reference documentation here or sign up for PropelAuth here.
Get ready to have an end-to-end identity solution for your app—without the usual headaches! We can’t wait to see what you build with PropelAuth for .NET.