Understanding Hydration Errors by building a SSR React Project
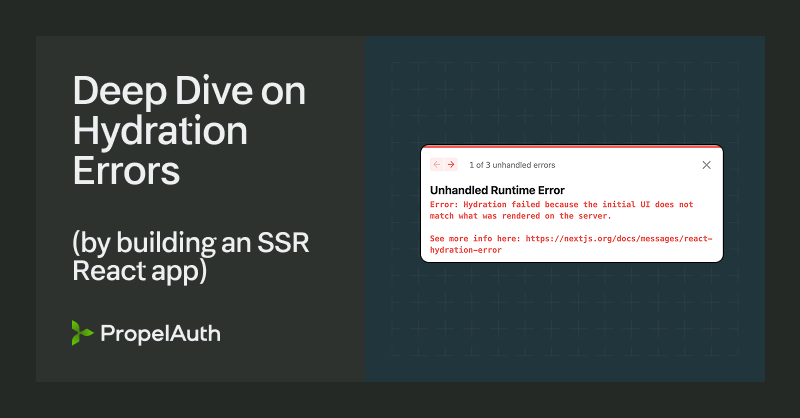
If you’ve written React code in any server-rendered framework, you’ve almost certainly gotten a hydration error. These look like:
Text content does not match server-rendered HTML
or
Error: Hydration failed because the initial UI does not match what was rendered on the server
And after the first time you see this, you quickly realize you can just dismiss it and move on... kind of odd for an error message that’s so in-your-face (later on, we’ll see that you might not want to dismiss them entirely).
So, what is a hydration error? And when should you care about them vs ignore them?
In this post, we’re going learn more about them by building a very simple React / Express App that uses server-side rendering.
But before we can answer that, we need to know what Server-Side Rendering is in the first place.
What is Server-Side Rendering?
Server-Side Rendering (SSR) is a technique where the server renders the HTML of a page before sending it to the client.
Historically, you’d find SSR applications commonly used along-side template engines like Jinja, Handlebars, or Thymeleaf (for all my Java friends out there) - which made the process of building applications like this simple.
We can contrast this with Client-Side Rendering (CSR) where the server sends a minimal HTML file and the majority of the work for rendering the page is done in javascript in the browser.
Building an example React SSR application
To start, we’ll install Express for our server and React:
npm install express react react-dom
Then, we’ll make a basic React component with a prop:
import React from 'react';
interface AppProps {
message: string;
}
function App({ message }: AppProps) {
return <div><h1>{message}</h1></div>
}
export default App;
Finally, we make an Express server that renders this component:
import express from 'express';
import React from 'react';
import { renderToString } from 'react-dom/server';
import App from './components/App';
const app = express();
const htmlTemplate = (reactHtml: string) => `
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>React SSR Example</title>
</head>
<body>
<div id="root">${reactHtml}</div>
</body>
</html>
`;
app.get('/', (req, res) => {
const message = 'Hello from the server!';
const appHtml = renderToString(React.createElement(App, { message }));
const fullPageHtml = htmlTemplate(appHtml)
res.send(fullPageHtml);
});
app.listen(3000, () => {
console.log(`Server running at http://localhost:3000`);
});
We run our server, navigate to http://localhost:3000, and we see that it worked:
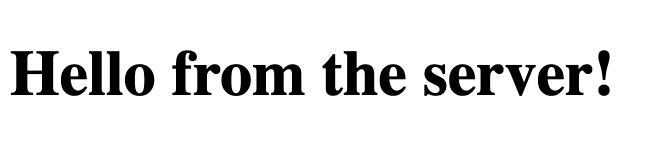
But, let’s see what happens when we add a counter to our component:
function App({ message }: AppProps) {
const [count, setCount] = React.useState(0)
return (
<div>
<h1>{message}</h1>
<p>Counter: {count}</p>
<button onClick={() => setCount(c => c+1)}>Increment</button>
</div>
);
}
It loads correctly, but clicking the button doesn’t do anything:
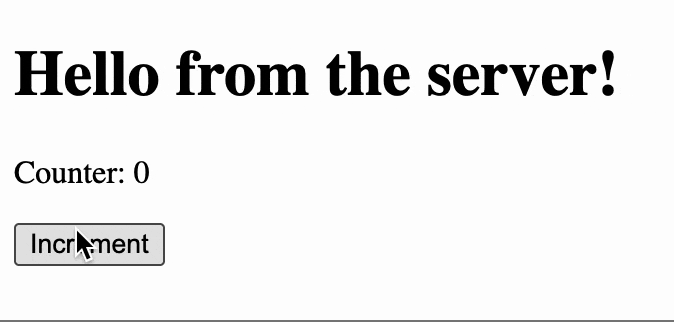
This is because renderToString
produces static HTML but doesn’t have any Javascript for handling events (like onClick
).
What we need is a way for the browser to attach event handlers and enable interactivity on top of server-rendered HTML - and that's what hydration does.
Hydrating our React Application
The key function here is hydrateRoot, whose description is:
hydrateRoot
lets you display React components inside a browser DOM node whose HTML content was previously generated byreact-dom/server
.
We can contrast that with createRoot, which you’ll find in CSR applications:
createRoot
lets you create a root to display React components inside a browser DOM node.
createRoot
assumes that it is setting up / displaying all the React components from scratch. hydrateRoot
assumes that it is setting up / displaying all the React components on top of our server rendered HTML.
If we look back on our htmlTemplate
, you can see that we are rendering our server HTML inside a div tag with an ID:
<div id="root">[server-rendered-html]</div>
So to “hydrate” our application, we just need to add some Javascript code on the client side, calling hydrateRoot
and referencing this div:
import React from 'react';
import { hydrateRoot } from 'react-dom/client';
import App from './components/App';
hydrateRoot(
document.getElementById('root'),
<App message="Hello from the server!" />
);
// Note that for this example, we're hard-coding the props
// But in a real application, we'd pass them down from the server
// One way to do this is to add a <script> tag that sets
// window.__INITIAL_PROPS__ = {"message": "Hello from the server!"}
// and then loads it here.
To make sure this runs, we’ll also need to update our template to add this script. We can add that underneath our <div id="root">${reactHtml}</div>
in our template:
<body>
<div id="root">${reactHtml}</div>
<script src="/bundle.js"></script>
</body>
For the purposes of not making this post too long, I am skipping over an important step here which is bundling our client entrypoint. For that you can use something like Vite or Rollup.
But, once we have that set up and we run our new code with hydrateRoot
, our counter now works:
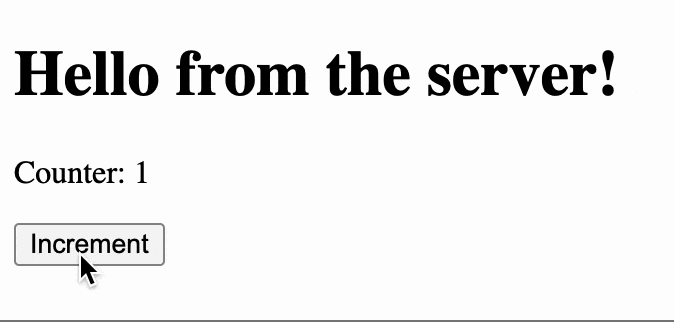
What happens when the client and server disagree?
Let’s take our example and make an obvious mistake. On the server, we’re passing in Hello from the server!
as a prop. What if the client instead passed in Hello from the client!
To make this more apparent, let’s also delay calling hydrateRoot
for a few seconds:
setTimeout(() => {
hydrateRoot(
document.getElementById('root'),
React.createElement(App, { message: 'Hello from the client!' })
)
}, 5000);
When we load the page, we initially see Hello from the server!
and then a few seconds later we get Hello from the client!
alongside a hydration error.
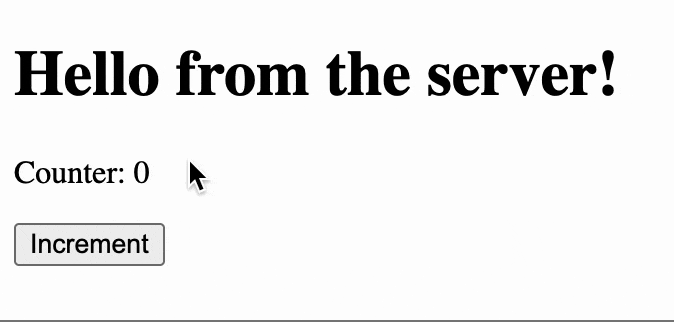
And ultimately that’s all a hydration error is - the server returned some HTML for a React component and when the client tried to load the same component, they didn’t match.
Why might you care about hydration errors?
One reason you may care about hydration errors is because it’s an awkward user experience. In our exaggerated example above, the page loaded with one message but the message completely changed a few seconds later.
For the more dangerous hydration errors, it’s worth thinking about how you might implement hydration yourself. The HTML for the component is already loaded, all you are trying to do is hook up event listeners to the right places.
What happens if the server returned something like:
<div>
<button onClick={deleteMyAccount}>Delete my account</button>
</div>
and the client sees something like:
<div>
<button>Upgrade my account</button>
<button>Delete my account</button>
</div>
Does the “Upgrade my account” button now trigger a delete (hopefully behind a confirmation modal) because it’s the first button under the div? Does neither button get the click handler? Do… both?
The reason to err on the side of caution here is because mismatched code can lead to some unfortunately ambiguous cases.
In practice, with mismatches like these, React will tear down and re-create the mismatched component tree to be safe, turning what could’ve been a correctness issue into a performance issue.
How do you get a hydration error in practice?
Obviously, the case where you pass in different props on the server vs the client is bound to lead to a hydration error.
One of the most straightforward, realistic examples is highlighted in the React docs here. If you need to render a timestamp, it’s possible for the server and client to disagree on the exact time.
Similarly, most things that check the window
or any browser-specific APIs can lead to these errors, since those only exist on the client and not the server.
One that I always found odd were nested p
tags. If you do something like:
<p>
<p>Text</p>
</p>
it also leads to a hydration error. The reason is actually pretty straightforward though, it’s because that isn’t actually valid HTML and the browser will correct it for you. Unfortunately for us, correcting it causes a mismatch between the client and server.
And unfortunately for me, this just highlights that I didn’t know that was invalid HTML.
Fixing hydration errors
At a high level, fixing hydration errors just means making sure the client and server match.
That’s really it. It’s going to be a bit different depending on what your code looks like, but you’ll want to think about what the server has access to vs what the browser has access to.
For that nested p
tag case, you need to make sure you are returning valid HTML so the browser doesn’t correct/modify it.
One notable pattern that you’ll find in StackOverflow posts is this isMounted
pattern:
const [isMounted, setIsMounted] = useState(false);
useEffect(() => {
setIsMounted(true);
}, []);
if (!isMounted) {
return null // alternatively return a placeholder
} else {
// do the thing you want to do
}
Why does this work?
Since useEffect blocks don't run until after hydration is complete, the only time isMounted
could be true is after hydration is finished, so both the client and server will see null
for this component.
And while this does fix the hydration error, it comes at the cost of… not really getting the benefits of SSR, since nothing is rendered on the client initially. But for smaller components or cases where a mismatch is unavoidable, this is one way to get rid of the error - you just likely don’t want to put this on your whole application.
Full example of fixing a hydration error
For a more concrete example, let’s say we make a hook like this:
const useSavedValue = () => {
const isBrowser = typeof window !== "undefined";
// We need to check if window is available, otherwise we'll get
// an error when we reference localStorage
const defaultSavedValue = isBrowser
? localStorage.getItem("savedValue") || "Default"
: "Default"
return useState(defaultSavedValue)
}
// Used in a component:
const Example = () => {
const [savedValue, setSavedValue] = useSavedValue()
return <pre>{savedValue}</pre>
}
Under what conditions (if any) would this Example
component cause a hydration error?
There’s three cases to think about:
On the server: isBrowser
is false, the Example
component will always render Default
On the client, with no value in localStorage: isBrowser
is true, defaultSavedValue
is Default
, so the component will always render Default
On the client, with “XYZ” in localStorage: isBrowser
is true, defaultSavedValue
is “XYZ”, so the component will render XYZ
This ends up being a hydration error that only occurs if you have a value other than “Default” saved in localStorage.getItem("savedValue")
. An especially annoying bug since different developers may or may not see it at all.
To fix this, we can rewrite our hook so that it always renders Default
, until hydration is complete:
const useSavedValue = () => {
const [value, setValue] = useState("Default");
// useEffect blocks don't run until after hydration is complete
useEffect(() => {
const savedValue = localStorage.getItem("savedValue");
if (savedValue) setValue(savedValue);
}, []);
return [value, setValue];
}
This also has the added benefit of not needing the isBrowser
check anymore, since useEffect
blocks don’t run on the server.
Fixing hydration errors is unfortunately going to be a little different depending on your code, but the most important thing to keep in mind is just “What renders on the server” vs “What renders on the client, before hydration.”
Summary
Hydration errors are an unfortunately common experience when you are writing React code in a lot of modern SSR frameworks.
Hydration errors occur when the HTML initially rendered by a server doesn't match the component structure React expects during client-side hydration.
Hopefully this post helped you understand a bit more about what hydration is in the first place, how those mismatches can occur, why they are ultimately problematic, and how to fix them.