FastAPI Auth with Dependency Injection
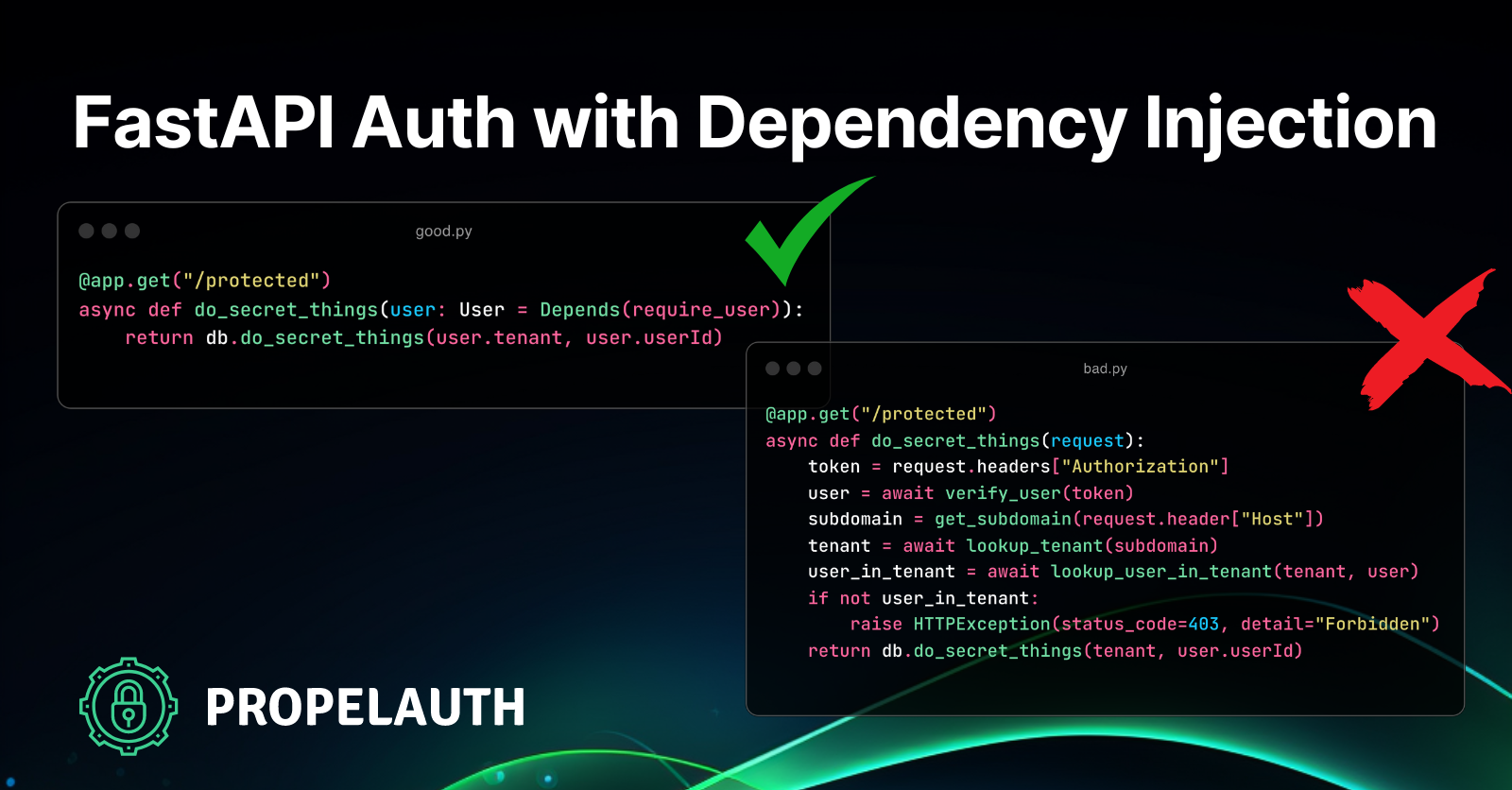
FastAPI is a modern web framework for building APIs in Python. It’s one of my personal favorite web frameworks as it has built-in support for OpenAPI specs (meaning you can write your backend code and generate everything from it) and it supports dependency injection.
In this post, we’ll briefly look at how FastAPI’s Depends works. We’ll then see why it applies so well to authentication and authorization. We’ll also contrast it with middleware, which is another common option for auth. Finally, we’ll look at some more advanced patterns for authorization in FastAPI.
What is Dependency Injection?
One of FastAPI’s more powerful features is its first class support for dependency injection. We have a longer guide here, but let’s look at a quick example of how it can be used.
Let’s say we are building a paginated API. Each API call may include a page_number
and a page_size
. Now, we could just create an API and take these parameters in directly:
@app.get("/things/")
async def fetch_things(page_number: int = 0, page_size: int = 100):
return db.fetch_things(page_number, page_size)
But, we probably want to add some validation logic so no one asks for page_number
-1 or page_size
10,000,000.
@app.get("/things/")
async def fetch_things(page_number: int = 0, page_size: int = 100):
if page_number < 0:
raise HTTPException(status_code=400, detail="Invalid page number")
elif page_size <= 0:
raise HTTPException(status_code=400, detail="Invalid page size")
elif page_size > 100:
raise HTTPException(status_code=400, detail="Page size can be at most 100")
return db.fetch_things(page_number, page_size)
And this is... fine, but if we had 10 APIs or 100 APIs that all needed the same paging params, it’d get a bit tedious. This is where dependency injection comes in - we can move all this logic into a function and inject that function into our API:
async def paging_params_dep(page_number: int = 0, page_size: int = 100):
if page_number < 0:
raise HTTPException(status_code=400, detail="Invalid page number")
elif page_size <= 0:
raise HTTPException(status_code=400, detail="Invalid page size")
elif page_size > 100:
raise HTTPException(status_code=400, detail="Page size can be at most 100")
return PagingParams(page_number, page_size)
@app.get("/things/")
async def fetch_things(paging_params: PagingParams = Depends(paging_params_dep)):
return db.fetch_things(paging_params)
@app.get("/other_things/")
async def fetch_other_things(paging_params: PagingParams = Depends(paging_params_dep)):
return db.fetch_other_things(paging_params)
This has some nice benefits:
- Each route that takes in
PagingParams
is automatically validated and has default values. - It’s less verbose and error-prone than having the first line of each route be
validate_paging_params(page_number, page_size)
- This still works with FastAPI’s OpenAPI support - those parameters will show up in your OpenAPI specs.
What does this have to do with authentication?
It turns out, this is also a great way to model auth! Imagine you had a function like:
async def validate_token(token: str):
try:
# This could be JWT validation, looking up a session token in the DB, etc.
return await get_user_for_token(token)
except:
return None
To hook this up to an API route, all we’d need to do is wrap it in a dependency:
async def require_valid_token_dep(req: Request):
# This could also be a cookie, x-api-key header, etc.
token = req.headers["Authorization"]
user = await validate_token(token)
if user == None:
raise HTTPException(status_code=401, detail="Unauthorized")
return user
And then all of our protected routes can add this dependency:
@app.get("/protected")
async def do_secret_things(user: User = Depends(require_valid_token_dep)):
# do something with the user
If the user provides a valid token, this route will run and user
is set. Otherwise, a 401 will be returned.
Note: OpenAPI/Swagger does have first-class support for specifying auth tokens, but you have to use one of the dedicated classes for it. Instead of req.headers["Authorization"]
, you can use HTTPBearer(auto_error=False)
from fastapi.security
which returns an HTTPAuthorizationCredentials
.
Middleware vs Depends for Auth
FastAPI, like most frameworks, has a concept of middleware. Your middleware can contain code that will run before and after a request. It can modify the request before the request gets to your route and it can modify the response before it’s returned to the user.
In many other frameworks, middleware is a really common place for authentication checks to take place. However, that’s often because the middleware is also tasked with “injecting” the user into the route. For example, a common pattern in Express is to do something like:
app.get("/protected", authMiddleware, (req, res) => {
// req.user is set by the middleware
// as there's no good way to pass in extra information into this route,
// outside of the request
});
Since FastAPI has a built-in concept of injection, you may not need to use middleware at all. I’d consider using middleware if you need to periodically “refresh” your auth tokens (to keep them alive) and set the response as a cookie.
In this case, you’ll want to use request.state
to pass information from the middleware to the routes (and you can use a dependency to validate the request.state
if you’d like).
Otherwise, I’d stick with using Depends as the user will be injected directly into your routes without needing to go through request.state
.
Authorization - Multi-tenancy, Roles & Permissions
If we apply everything we learned so far, adding in multi-tenancy, roles or permissions can be pretty straightforward. Let’s say we have a unique subdomain for each of our customers, we can make a dependency for this subdomain:
async def tenant_by_subdomain_dep(request: Request) -> Optional[str]:
# first we get the subdomain from the host header
host = request.headers.get("host", "")
parts = host.split(".")
if len(parts) <= 2:
raise HTTPException(status_code=404, detail="Not found")
subdomain = parts[0]
# then we lookup the tenant by subdomain
tenant = await lookup_tenant_for_subdomain(subdomain)
if tenant == None:
raise HTTPException(status_code=404, detail="Not found")
return tenant
We can combine this idea with our previous ideas and make a new “multi-tenant” dependency:
async def get_user_and_tenant_for_token(
user: User = Depends(require_valid_token_dep),
tenant: Tenant = Depends(tenant_by_subdomain_dep),
) -> UserAndTenant:
is_user_in_tenant = await check_user_is_in_tenant(tenant, user)
if is_user_in_tenant:
return UserAndTenant(user, tenant)
raise HTTPException(status_code=403, detail="Forbidden")
We can then inject this dependency into our routes:
@app.get("/protected")
async def do_secret_things(user_and_tenant: UserAndTenant = Depends(get_user_and_tenant_for_token)):
# do something with the user and tenant
And this ends up doing a few major things:
- Checking that the user has a valid token
- Checking that the user is making a request to a valid subdomain
- Checking that the user should have access to that subdomain
If any of those invariants aren’t met - an error is returned and our route will never run. We can extend this to include other things like roles & permissions (RBAC) or making sure the user has a certain property set (active paid subscription vs no active subscription).
PropelAuth <3 FastAPI
At PropelAuth, we’re big fans of FastAPI. We have a FastAPI library that will enable you to set up authentication and authorization quickly - including SSO, Enterprise SSO / SAML, SCIM Provisioning, and more.
And it all works with dependencies like the ones you’ve seen above, e.g.:
@app.get("/")
async def root(current_user: User = Depends(auth.require_user)):
return {"message": f"Hello {current_user.user_id}"}
You can find out more here.
Summary
- FastAPI's dependency injection provides a powerful way to handle authentication and authorization in web applications.
- The
Depends
feature allows for clean, reusable code for validating tokens, checking user permissions, and handling multi-tenancy. - Compared to middleware, using dependencies for auth offers more flexibility and direct integration with route functions.
- Complex authorization scenarios like multi-tenancy and role-based access control can be efficiently implemented using nested dependencies.
- PropelAuth offers a FastAPI library that simplifies the implementation of advanced authentication and authorization features.